What is Beans and Dependency Injection in Spring Boot
In the ever-evolving landscape of software development, Spring Boot has emerged as a prominent framework for building Java-based applications. It offers a streamlined and efficient way to create robust and scalable applications. Two fundamental concepts at the core of Spring Boot's architecture are "Beans" and "Dependency Injection." In this article, we will delve deep into these concepts, exploring what they are, how they work, and why they are crucial in Spring Boot development.
Table of Contents
- Introduction to Spring Boot
- Understanding Beans
- What are Beans?
- Why are Beans Important?
- Creating Beans in Spring Boot
- The Essence of Dependency Injection
- What is Dependency Injection?
- Types of Dependency Injection
- How Spring Boot Implements Dependency Injection
- Benefits of Using Dependency Injection
- Spring Boot's Dependency Injection in Action
- Constructor Injection
- Setter Injection
- Field Injection
- Managing Beans and Their Scopes
- Singleton Scope
- Prototype Scope
- Request and Session Scopes
- Resolving Dependencies
- Autowiring
- Qualifiers
- Best Practices for Bean and Dependency Injection
- Common Pitfalls to Avoid
- Real-World Examples
- Integrating Beans and Dependency Injection into a Spring Boot Application
- Performance Considerations
- Evolving Your Spring Boot Application
- Future Trends and Developments
- Conclusion
1. Introduction to Spring Boot
Spring Boot is a Java-based framework that simplifies the process of building production-ready applications. It provides pre-configured templates and conventions for application development, reducing the need for extensive boilerplate code. At the heart of Spring Boot lies the concept of "Beans" and "Dependency Injection," which make it easier to manage and scale your application components.
2. Understanding Beans
2.1. What are Beans?
In Spring Boot, a "Bean" is a managed object within the application context. These objects are created, assembled, and managed by the Spring IoC (Inversion of Control) container. Beans represent the various components of your application, such as services, repositories, controllers, and more.
2.2. Why are Beans Important?
Beans are vital because they promote modularity and reusability in your code. By defining your application's components as beans, you can easily wire them together and manage their lifecycle. This decoupling of components simplifies testing, maintenance, and future updates.
2.3. Creating Beans in Spring Boot
Creating a bean in Spring Boot is straightforward. You can use annotations like @Component
, @Service
, or @Repository
to mark a class as a bean. The Spring IoC container takes care of instantiating and configuring these beans, making them available for dependency injection.
3. The Essence of Dependency Injection
3.1. What is Dependency Injection?
Dependency Injection (DI) is a design pattern used in Spring Boot to achieve loose coupling between classes. It involves injecting the required dependencies (usually beans) into a class rather than having the class create them itself. This approach promotes code reusability and testability.
3.2. Types of Dependency Injection
In Spring Boot, there are three primary ways to perform dependency injection:
- Constructor Injection: Dependencies are injected through the constructor of a class.
- Setter Injection: Dependencies are injected using setter methods.
- Field Injection: Dependencies are injected directly into fields of a class.
3.3. How Spring Boot Implements Dependency Injection
Spring Boot uses its IoC container to manage and inject dependencies. By annotating the fields, constructors, or setter methods with @Autowired
, you can indicate where Spring should inject the required dependencies.
4. Benefits of Using Dependency Injection
Dependency Injection offers several advantages:
- Flexibility: You can easily replace or update dependencies without altering the class using them.
- Testability: It simplifies unit testing by allowing you to provide mock dependencies.
- Maintainability: Code becomes more modular and easier to maintain.
- Scalability: As your application grows, managing dependencies becomes more manageable.
5. Spring Boot's Dependency Injection in Action
5.1. Constructor Injection
Constructor injection is considered a best practice in Spring Boot. It ensures that all required dependencies are provided when an object is created.
5.2. Setter Injection
Setter injection allows you to set dependencies after object creation. It offers flexibility but can lead to instances with incomplete dependencies if not used carefully.
5.3. Field Injection
Field injection injects dependencies directly into class fields. While convenient, it can make it harder to identify a class's dependencies at a glance.
6. Managing Beans and Their Scopes
6.1. Singleton Scope
By default, Spring Boot beans are singletons, meaning there is only one instance of a bean per application context.
6.2. Prototype Scope
Beans with prototype scope are created each time they are requested. This is useful for stateful components.
6.3. Request and Session Scopes
In web applications, you can use request and session scopes to manage beans specific to HTTP requests and sessions.
7. Resolving Dependencies
7.1. Autowiring
Autowiring in Spring Boot automatically resolves dependencies by type. It simplifies configuration but may lead to ambiguity in complex scenarios.
7.2. Qualifiers
Qualifiers help disambiguate when multiple beans of the same type exist, allowing you to specify which one to inject.
8. Best Practices for Bean and Dependency Injection
To ensure clean and maintainable code, adhere to best practices like using constructor injection, providing clear bean names, and using qualifiers when necessary.
9. Common Pitfalls to Avoid
Avoid common pitfalls such as circular dependencies and overusing field injection to maintain the integrity of your Spring Boot application.
10. Real-World Examples
Explore real-world examples of how Beans and Dependency Injection are used in Spring Boot applications.
11. Integrating Beans and Dependency Injection into a Spring Boot Application
Learn how to integrate these concepts into your Spring Boot project, allowing you to harness their full potential.
12. Performance Considerations
Consider the performance implications of Beans and Dependency Injection in your application and optimize where necessary.
13. Evolving Your Spring Boot Application
As your application evolves, adapt your Bean and Dependency Injection strategies to meet changing requirements.
14. Future Trends and Developments
Stay informed about emerging trends and developments related to Spring Boot and dependency management in Java.
Beans and Dependency Injection in Spring Boot
Let's understand first here that what is a bean in spring and how did we use the IOC in spring framework?
What is Beans in Spring?
The objects that form the backbone of your application
The objects that are managed by the Spring IoC container are called beans.
A bean is an object that is instantiated, assembled, and otherwise managed by a Spring IoC container.
A bean is simply one of many objects in your application.
Let's see in the application, how beans get initialized for spring application:
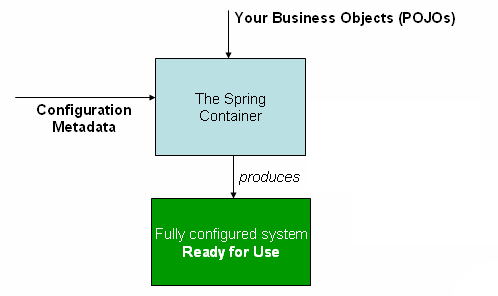
Beans in Spring Boot
The @ComponentScan annotation is used to find beans in spring framework.
These beans are being injected with @Autowired annotation.
But, there is no need to specify any arguments for @ComponentScan annotation in the Spring Boot.
All component class files are registered with Spring Beans automatically.
This is very easy example to understand the auto-wiring the Rest Template object and creating a Bean for the same
package com.techieuncle.app;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
public class ExampleApplication {
@Autowired
RestTemplate restTemplate;
public static void main(String[] args) {
SpringApplication.run(ExampleApplication.class, args);
}
@Bean
public RestTemplate findRestTemplate() {
return new RestTemplate();
}
}
15. Conclusion
In conclusion, Beans and Dependency Injection are foundational concepts in Spring Boot development. They promote modular, maintainable, and scalable...